API (Application Programming Interface) integration is essential for modern web development, allowing your web projects to communicate with external services and enhance functionality. This guide covers the key steps for implementing API integration effectively in your web projects.
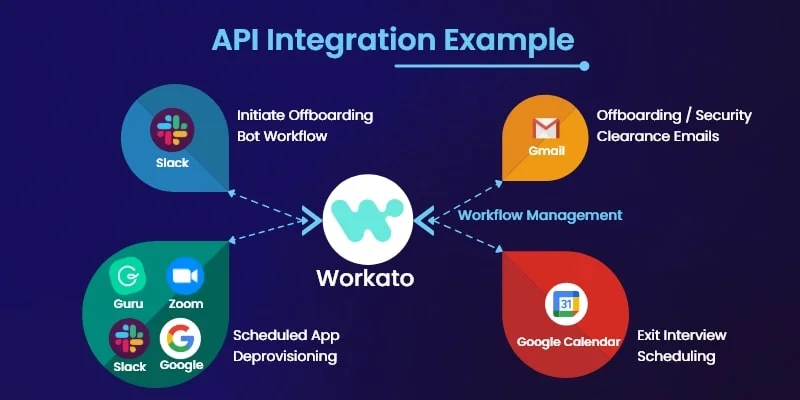
1. Understanding APIs
APIs are interfaces that enable different software applications to interact with each other. They define the methods and data formats for communication, making it possible to access and use external services and data.
- Types of APIs: Common API types include REST (Representational State Transfer), SOAP (Simple Object Access Protocol), and GraphQL. REST is the most widely used for web applications due to its simplicity and compatibility with HTTP.
- API Endpoints: An API endpoint is a specific URL where API requests are sent. Each endpoint corresponds to a specific function or resource.
2. Choosing the Right API
Selecting the right API for your project involves evaluating several factors.
- API Documentation: Review the API’s documentation to understand its functionality, endpoints, and authentication methods. Good documentation is crucial for smooth integration.
- Rate Limits and Quotas: Check the API’s rate limits and usage quotas to ensure it meets your project’s needs without exceeding usage limits.
- Security: Ensure the API supports secure communication (e.g., HTTPS) and provides authentication mechanisms (e.g., API keys, OAuth).
3. Setting Up API Integration
3.1. Obtain API Credentials
- API Key: Most APIs require an API key for authentication. Sign up for the API service and obtain your API key from the provider’s dashboard.
- OAuth Tokens: Some APIs use OAuth for authentication. Follow the provider’s instructions to obtain and manage OAuth tokens.
3.2. Make API Requests
- Choose a Method: Determine the HTTP method for your request (e.g., GET for fetching data, POST for sending data).
- Send Requests: Use tools or libraries to send API requests. In JavaScript, you can use the
fetch
API or libraries like Axios.javascript
// Using fetch API
fetch('https://api.example.com/data', {
method: 'GET',
headers: {
'Authorization': 'Bearer YOUR_API_KEY'
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
javascript
// Using Axios
axios.get('https://api.example.com/data', {
headers: {
'Authorization': 'Bearer YOUR_API_KEY'
}
})
.then(response => console.log(response.data))
.catch(error => console.error('Error:', error));
3.3. Handle API Responses
- Process Data: Parse and process the data returned by the API. Ensure you handle errors and edge cases, such as empty responses or server errors.
- Update UI: Use the data to update your web application’s UI dynamically. For example, display fetched data in a table or populate a form.
4. Error Handling and Debugging
Proper error handling ensures a smooth user experience and helps you diagnose issues.
- Check Status Codes: Inspect HTTP status codes in responses to determine the success or failure of requests. Handle errors based on status codes (e.g., 400 for client errors, 500 for server errors).
- Log Errors: Implement logging to capture and analyze errors. This helps in debugging and improving the integration.
javascript
fetch('https://api.example.com/data')
.then(response => {
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
return response.json();
})
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
5. Optimize API Usage
Efficient API usage improves performance and reduces costs.
- Caching: Implement caching strategies to reduce the number of API requests and speed up data retrieval. Use browser caching or server-side caching.
- Throttle Requests: Respect API rate limits by throttling your requests. Use techniques like batching or queuing to manage request frequency.
- Minimize Data: Request only the data you need by specifying fields or using query parameters. This reduces payload size and improves performance.
6. Ensure Security
Security is critical when integrating with APIs to protect sensitive data and maintain application integrity.
- Use HTTPS: Ensure all API communications are encrypted with HTTPS to protect data in transit.
- Secure API Keys: Keep API keys and tokens secure. Avoid hardcoding them in your codebase. Use environment variables or secure storage solutions.
- Validate Input: Validate and sanitize user inputs before sending them to the API to prevent security vulnerabilities such as SQL injection or cross-site scripting (XSS).
7. Documentation and Maintenance
Good documentation and regular maintenance keep your API integration efficient and manageable.
- Document Integration: Document your API integration process, including endpoints, request formats, and response handling. This helps in future maintenance and team collaboration.
- Monitor Usage: Monitor API usage and performance to identify potential issues or improvements. Regularly review and update your integration as needed.
Conclusion
Implementing API integration in your web projects involves selecting the right API, setting up requests, handling responses, and ensuring security. By following best practices for error handling, optimization, and security, you can create robust and efficient integrations that enhance your web applications’ functionality and user experience.